To run Powershell cmdlet’s from C#, we will use this namespace: Microsoft.PowerShell.SDK
There are a few code preparations we need to to able to run PowerCLI from Linux. If you set the execution policy like below, you need to aware that this piece can only run on Windows.
PowerShell powershell = PowerShell.Create();
Command setExecutionPolicy = new Command("Set-ExecutionPolicy");
setExecutionPolicy.Parameters.Add("ExecutionPolicy", "Unrestricted");
setExecutionPolicy.Parameters.Add("Force");
powershell.Commands.AddCommand(setExecutionPolicy);
powershell.Invoke();
So for Linux we need to add the following check
PowerShell powershell = PowerShell.Create();
if (RuntimeInformation.IsOSPlatform(OSPlatform.Windows))
{
Command setExecutionPolicy = new Command("Set-ExecutionPolicy");
setExecutionPolicy.Parameters.Add("ExecutionPolicy", "Unrestricted");
setExecutionPolicy.Parameters.Add("Force");
powershell.Commands.AddCommand(setExecutionPolicy);
powershell.Invoke();
}
To able to run PowerCLI cmdlet’s, we need to connect to at least one vCenter server like following
// Import Module
powershell = PowerShell.Create();
powershell.Runspace = runspace;
Command importModule = new Command("Import-Module");
importModule.Parameters.Add("Name", "VMware.VimAutomation.Core");
importModule.Parameters.Add("Force");
powershell.Commands.AddCommand(importModule);
powershell.Invoke();
// Set PowerCLI config
powershell = PowerShell.Create();
powershell.Runspace = runspace;
Command setPowerCLIConfig = new Command("Set-PowerCLIConfiguration");
setPowerCLIConfig.Parameters.Add("Scope", "User");
setPowerCLIConfig.Parameters.Add("ParticipateInCEIP", false);
setPowerCLIConfig.Parameters.Add("InvalidCertificateAction", "Ignore");
setPowerCLIConfig.Parameters.Add("Confirm", false);
setPowerCLIConfig.Parameters.Add("DefaultVIServerMode", "Single");
setPowerCLIConfig.Parameters.Add("WebOperationTimeoutSeconds", -1);
powershell.Commands.AddCommand(setPowerCLIConfig);
powershell.Invoke();
// Connect VI-Server
powershell = PowerShell.Create();
powershell.Runspace = runspace;
Command connectVI = new Command("Connect-VIServer");
connectVI.Parameters.Add("Server", vcenter);
connectVI.Parameters.Add("User", username);
connectVI.Parameters.Add("Password", password);
connectVI.Parameters.Add("Force");
powershell.Commands.AddCommand(connectVI);
powershell.Invoke();
Now the vCenter connection is established and we can do the action we need on the vCenter.
To be able to run this within a Linux container, add the following dockerfile. In this case we set the Environment variable to development since I will run this within Docker desktop / Aspire.
# Use .NET 9 images
FROM mcr.microsoft.com/dotnet/sdk:9.0 AS base
WORKDIR /app
# Install PowerShell + PowerCLI
RUN apt-get update && \
apt-get install -y wget apt-transport-https software-properties-common && \
wget -q "https://packages.microsoft.com/config/debian/11/packages-microsoft-prod.deb" && \
dpkg -i packages-microsoft-prod.deb && \
apt-get update && \
apt-get install -y powershell && \
pwsh -Command "Install-Module -Name VMware.PowerCLI -Scope AllUsers -Force -AllowClobber"
# Copy the published output
COPY . .
ENV DOTNET_ENVIRONMENT=Development
# Define the entry point for the application
ENTRYPOINT ["dotnet", "VMwareWorker.dll"]
Now built and deploy the container.
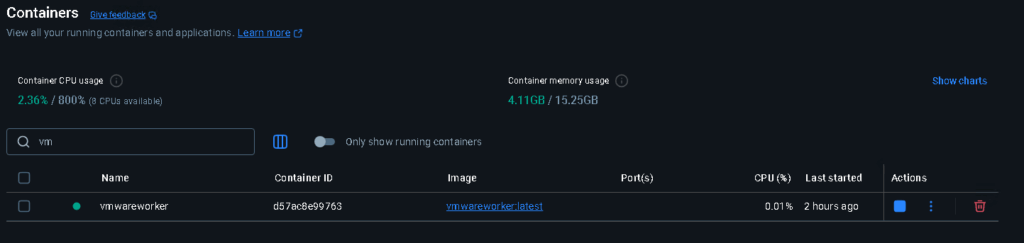